Code: Select all
import ptsxpy as p
cb1 = p.CreateCube( 1, 2., 2., 2. )
p.SceneAddObject( cb1, e_tsxFALSE )
p.SceneDraw()
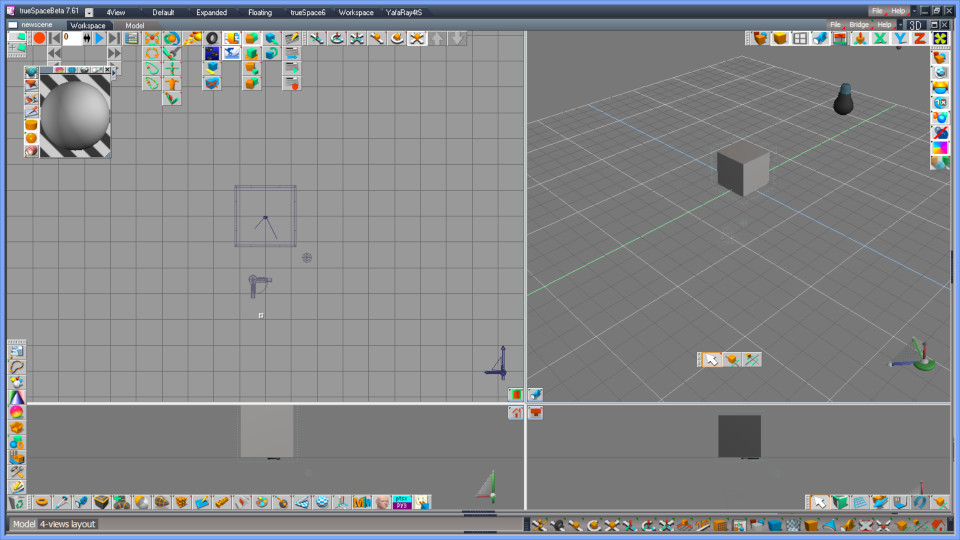
In the first line, the script declares to use a module "ptsxpy" and applies an alias "p" to it.
Code: Select all
import ptsxpy as p
moduleoralias.method( parameter1, parameter2, ...)
In this case, we use ptsxpy or p for moduleoralias.
Code: Select all
cb1 = p.CreateCube( 1, 2., 2., 2. )
p.SceneAddObject( cb1, e_tsxFALSE )
p.SceneDraw()
You should be able to get the official SDK that includes "tsxAPI.doc" via the net though it has not been supported by the vender(s). Please note that we should refer not "TSXPYTHON.DOC" (titled "Caligari tsxPython Interface") but tsxAPIxx.doc (where xx is code for each tS version, and titled "Caligari trueSpace eXtension") in order to develop your python scripts for ptsxpy.
In order to add and display a cube to a scene, we use functions below;
- CreateCube() .... corresponds to tsxCreateCube() in tsxAPI
- SceneAddObject() .... corresponds to tsxSceneAddObject() in tsxAPI
- SceneDraw() .... corresponds to tsxSceneDraw() in tsxAPI
Code: Select all
cb1 = p.CreateCube( 1, 2., 2., 2. )
- tsxPOLYHEDRON* tsxCreateCube(int nbrSections,float x,float y,float z)
In this code example, we assign (i.e. copy) the returned value to a variable "cb1". Of course, you can freely name it (according to the naming rule of Python language). We can use the variable in the following lines. In other words, we must assigned the returned value to a variable in order to use the created object.
Please note and keep in mind that the byte length of a pointer is 4 (i.e. 32 bit) and equivalent to unsigned long type without any exception for all tS versions. In other words, the tsxCreateCube() returns an integer value 0 thru [latexin]2^{32}[/latexin]-1 (0x00000000 thru 0xffffffff in hexadecimal). It's the same in case of ptsxpy; CreateCube() returns an integer value and cb1 is to have the value. If you would like to know the value, add print() like;
Code: Select all
cb1 = p.CreateCube( 1, 2., 2., 2. )
print( "cb1=", cb1)
Code: Select all
cb1 = p.CreateCube( 1, 2., 2., 2. )
print( "cb1=0x%08x" % cb1)
Now let's take a look at the parameters of CreateCube(). The first parameter nbrSections is defined as "resolution" in the tsxAPIXX.doc. Specifying 1 to it means the cube to be created does not have any latitude and longitude other than its minimally required twelve edges (You can see what is latitude and longitude if you change it to 2 or 3). The second, third, and fourth parameters determines each dimension (size) of x, y, z. Please try to change and look how the result changes. Note that each of x, y, z must be a floating point value (you can omit the 0 of 1.0).
Code: Select all
p.SceneAddObject( cb1, e_tsxFALSE )
p.SceneDraw()
- void tsxSceneAddObject(tsxGNODE* pGNode,tsxBOOL bNoDraw)
- pGNode tsxGNODE*: the object to be added
- bNoDraw tsxBOOL: TRUE if the new object shouldn't be drawn within this function call
- enum tsxBOOL { e_tsxFALSE = 0, e_tsxTRUE };
Code: Select all
e_tsxFALSE = 0
e_tsxTRUE = 1
Until this SceneAddObject() is called, the cube stays invisible (though some manipulation actions like move or scale can be done before visualize it). The SceneDraw() refreshes the view. Even in case you omit it, you can refresh the view by hand using View Control, etc.
Please note that calling SceneAddObject() is needed for each object and calling SceneDraw() once at the end of the script is enough.